Introduction to REST APIs in GoFiber
Mon Mar 18 2024
Views: Loading...
An introduction to the Go web framework, GoFiber.
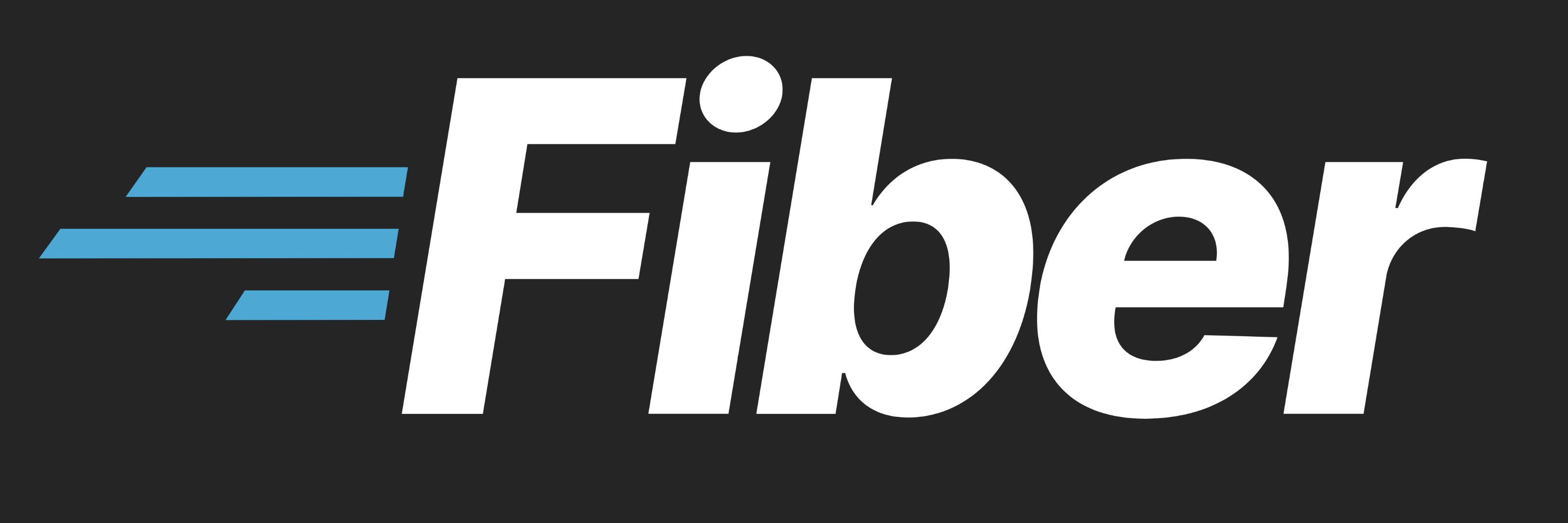
Introduction#
What is GoFiber?#
GoFiber is an open-source web framework built in the Go programming language. It is one of the fastest Go web frameworks and has over 30k stars on GitHub.
What is a REST API?#
A RESTful API is simply a defined way for sending and receiving information between two computers over the internet using HTTP requests and responses. You can learn more about REST API’s through Google’s About RESTful API’s page.
Why should I use GoFiber for building RESTful APIs?#
GoFiber is a perfect for REST API projects that are running on resource-constrained devices, as it was built with zero memory allocation and performance in mind.
GoFiber is also well-documented and has a active discord community, where you can ask any questions if you run into any issues while using the framework.
Prerequisites#
GoFiber requires Go version 1.21 or higher to run. Please follow the official go.dev installation instructions to install the latest Go compiler.
Tutorial#
Step 1. Install Go Fiber#
Before getting started, you must get the GoFiber package to import into your project. To download the package, please run the following:
# Create a directory/folder called "my-api" and
# change directories into it
mkdir my-api
cd my-api
# Initialize a Go module called `my-api`
# (making a module is required to install packages)
go mod init my-api
# Install GoFiber v2
go get -u github.com/gofiber/fiber/v2
💡 Note: As of March 18, 2024, the latest stable version of GoFiber is v2, with v3 currently in development. This tutorial will use version 2.52.2 from this point onward.
Step 2. Create a new file in the my-api/
folder and call it my_server.go
#
The file my_server.go
will be the main file for our
GoFiber REST API.
In the file, type in the following:
package main
import (
"fmt"
"github.com/gofiber/fiber/v2"
)
func main() {
// Create a new GoFiber application
app := fiber.New(fiber.Config{})
// TODO: Write our first API endpoint
// ==================================
// Start listening for REST API Requests
err := app.Listen(":8000")
// If an error occurs, print out the error
// and quit
if err != nil {
fmt.Println("Error:", err)
}
}
This is the basic scaffolding for every GoFiber API.
If you were to run this as-is, you would have a
working server that’s running at 127.0.0.1:8000/
.
Since we haven’t defined anything besides starting the GoFiber server, the server currently doesn’t do anything.
So let’s make it do something!
Step 3. Add a GET endpoint for “/”#
Add the following to the main function under the // TODO
comment from before:
// ...
func main() {
// ...
// TODO: Write our first API endpoint
// ==================================
app.Get("/", func (c *fiber.Ctx) error {
return c.JSON(fiber.Map{
"message": "Hello World!",
})
})
// ...
}
This code will add logic that will run when you
navigate to 127.0.0.1:8000/
, which is the GET "/"
endpoint.
💡 Note: When you navigate to a link (like
127.0.0.1:8000/
), your browser makes aGET
request by default to the link.
Step 4. Run the server#
Let’s now run the server and see what happens:
While inside of the my-api
folder, compile
my_server.go
and run the program:
# Compile my_server.go
go build -o my_server my_server.go
# Run the executable (MacOS/Linux)
./my_server
# Or Run the executable (Windows)
my_server.exe
You should get the following output:
Step 5. Visit your GET “/” endpoint in the browser#
Now, open a new tab in your favorite browser and type
into the address bar: 127.0.0.1:8000/
You should see the following on your screen:
{"message":"Hello World!"}
For example, on Safari:
Congratulations, you just built your first RESTful API in GoFiber!
Next Steps#
Don’t stop learning about GoFiber here. You can explore its documentation here and find out what GoFiber offers.
If you would like me to write more blogs about GoFiber, feel free to contact me and let me know you would like to see more of these.
Happy learning!